Creating API Using Python Flask And MongoDB Client
- realcode4you
- Sep 12, 2021
- 2 min read
First Import All Related Libraries
#Import Libraries
from flask import Flask
from pymongo import MongoClient
from flask import request
import json
from bson.json_util import dumps
Creating Connection With MongoDB Client
client = MongoClient('localhost:27017')
db = client.market
Implementation
@app.route("/")
def hello():
return "Ok it works"
@app.route('/stock', methods=['GET', 'POST', 'PUT', 'DELETE'])
def stock():
if request.method=='GET':
try:
result = db.stocks.find()
return dumps(result)
except Exception:
return dumps({'error' : str("error")})
elif request.method=='POST':
try:
result = db.stocks.insert({"Ticker":"XYZ"})
return dumps(result)
except Exception:
return dumps({'error' : str("error")})
elif request.method=='PUT':
try:
result = db.stocks.updateMany({"Ticker":"AAMC"},{'$set':{"Ticker":"ABC"}})
return dumps(result)
except Exception:
return dumps({'error' : str("error")})
elif request.method=='DELETE':
try:
delete_user = db.stocks.delete_one({"Ticker":"ABC"})
if delete_user.deleted_count > 0 :
return "", 204
else:
return "", 404
except:
return "", 500
else:
return("ok")
if __name__ == "__main__":
app.run()
Follow Below Steps Before Run Above Code
step first:
open cmd prompt
step second:
----------------------------
>mkdir rest
>cd rest
----------------------------
step third
----------------------------------
check rest folder created copy path and run command as per screenshot 3
step fourth
----------------------------------------
C:\Users\naveen kumar\rest>virtualenv flask
installation start
script and other folder are created
step fifth
----------------------------------------
again go to the rest folder and copy path where script folder exist
then activate virtual environment by activate command
C:\Users\naveen kumar\rest\flask\Scripts>activate
step six
------------------------------------------
Create python file using any editor but save it into the scipt folder
-------------------------------------------------------
step seven
Now install flask by using given command and see in screeshot
(flask) C:\Users\naveen kumar\rest\flask\Scripts>pip install flask
-------------------------------------------
step eight
Now intall pymongo by using command
(flask) C:\Users\naveen kumar\rest\flask\Scripts>pip install pymongo
step ninth
run python file using command line(sure python file is open in editor)
(flask) C:\Users\naveen kumar\rest\flask\Scripts>python restfullapiflask.py
url is generated on command line
-------------------------------------------
step ten
copy url and paste it into webbrowser like chrome and run
as per screenshot 8
and you see the output -----Ok it works
-------------------------------------------
now start mongod and mongo
and import json file which you already complete this
-----------------------------------------
install postman and run url http://127.0.0.1:5000/stoks
as per get, put and delete
find output result
thanks
---------------------------------------------------
Get
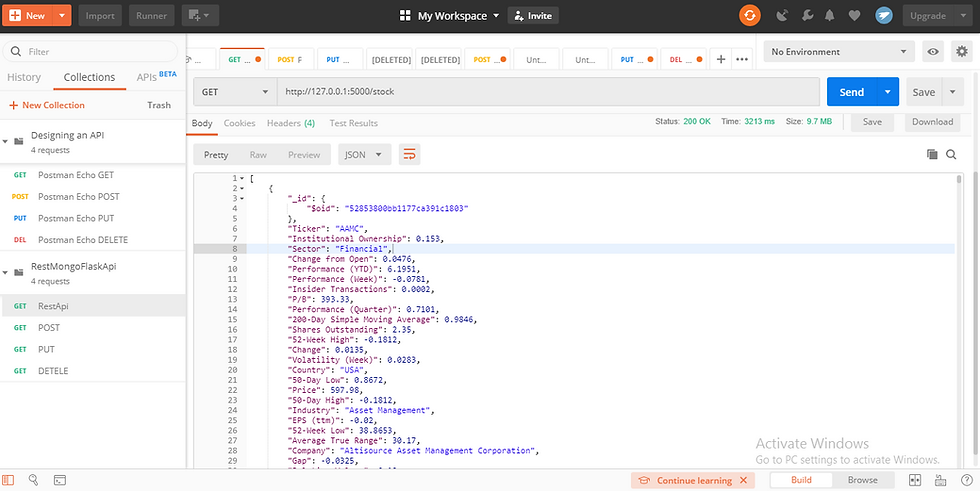
Post

Delete
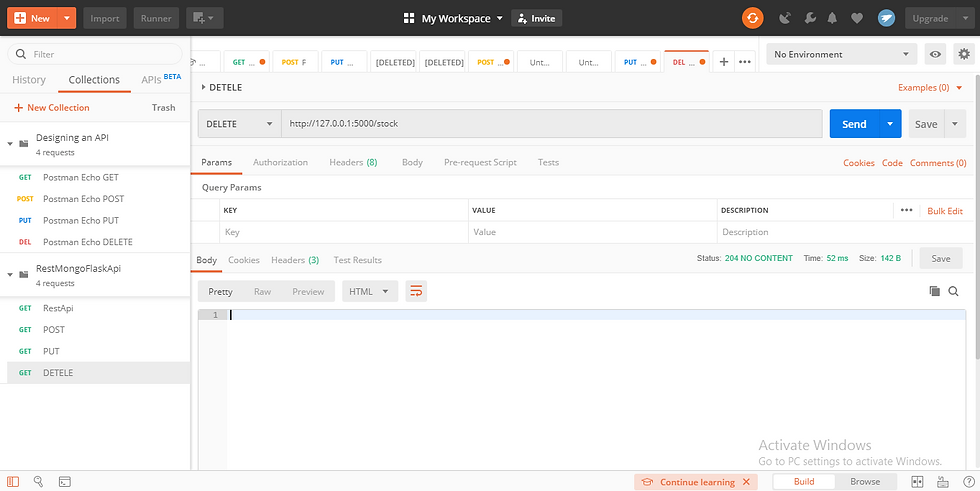
If you need Other Help Related to Python then contact us or send your project requirement details at realcode4you@gmail.com and get instant help with an affordable price.
Comments