Develop Web Form Using HTML, CSS | Get Help In HTML, CSS And JavaScript Projects
- realcode4you
- Jul 16, 2021
- 5 min read
Introduction
Using HTML and CSS to develop web forms. You only need to build the front-end web forms. There is no back-end server services, so when the forms are submitted, it will fail. You should use CSS and HTML to make your forms look presentable.
Web Form 1
As per below screenshot here we have write the HTML, CSS code to create this web form:
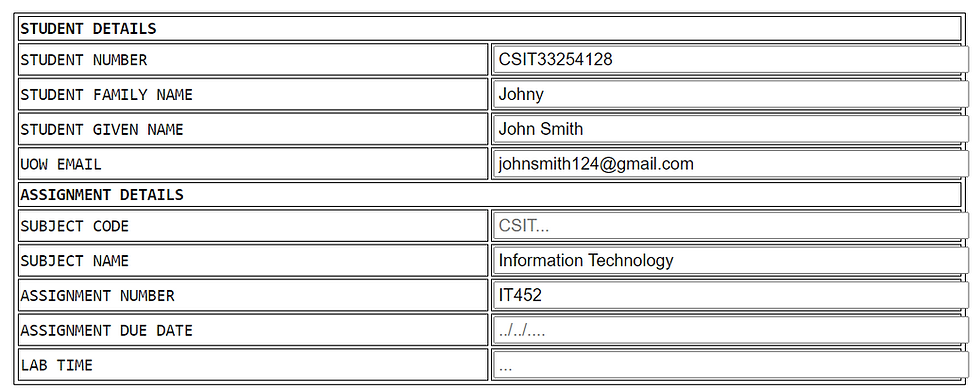
Script:
<!DOCTYPE html>
<html>
<head>
<title>Form 1</title>
<style>
body{
font-family: monospace;
}
.container {
padding-right: 20%;
padding-left: 20%;
margin-right: auto;
margin-left: auto;
}
.outer{
margin-right: -15px;
margin-left: -15px;
display: inline-block;
width: 100%;
}
.outer.bg-blue{
background-color: #131365;
}
.inner-50{
width: 50%;
float: left;
padding-left: 15px;
padding-right: 15px;
box-sizing: border-box;
}
.inner-50.left{
background-color: #a4c9e7;
}
.inner-50.right{
background-color: #131365;
color: white;
}
.inner-50 h2{
text-align: center;
}
.inner-50 .checkbox label input{
margin-right:10px;
}
.inner-50 .checkbox label{
font-weight: 600;
width: 100%;
display: inline-block;
}
.inner-50 small{
padding-left: 30px;
display: inline-block;
color: #514f4f;
}
.hr-bold{
font-weight: 600;
}
.group .control{
margin-top: 5px;
width: 100%;
height: 34px;
background-color: #fff;
background-image: none;
border: 1px solid #ccc;
border-radius: 4px;
margin-bottom: 20px;
}
.group textarea {
margin-top: 5px;
width: 100%;
background-color: #fff;
background-image: none;
border: 1px solid #ccc;
border-radius: 4px;
margin-bottom: 20px;
}
.group select{
width: auto !important;
}
.inner-group{
width: 100%;
display: inline-block;
text-align: left;
}
.stacked-group{
float: left;
width: 38%;
display: inline-block;
margin-right: 10px;
box-sizing: border-box;
}
.btn-success{
color: #080808;
border-radius: 5px;
display: inline-block;
padding: 6px 12px;
margin-bottom: 0;
font-size: 14px;
font-weight: 400;
line-height: 1.42857143;
text-align: center;
white-space: nowrap;
vertical-align: middle;
-ms-touch-action: manipulation;
touch-action: manipulation;
cursor: pointer;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
background-image: none;
border: 1px solid transparent;
border-radius: 4px;
}
.btn-success.grey{
background-color: #c1c1c1;
}
.btn-success.cyan{
background-color: #0fb3b3;
color: white;
}
.checkbox-label{
display: inline-block;
vertical-align: middle;
}
.text-center{
text-align: center;
}
table{
width: 100%;
border: 1px solid black;
}
table tr th, table tr td{
border: 1px solid black;
text-align:left;
}
table tr td input{
width: 100%;
}
</style>
</head>
<body>
<div class="container">
<h1><b>Assignment 2 Solution</b></h1>
<hr>
<h3>I have read the policy of plagiarism at Wollongong University.</h3>
<h3>I declare that this assinment is entirely my work.</h3>
<hr>
<h1><b>Part 1</b></h1>
<table>
<colgroup>
<col style="width:50%">
<col style="width:50%">
</colgroup>
<tbody>
<tr>
<th colspan="2">STUDENT DETAILS</th>
</tr>
<tr>
<td>STUDENT NUMBER</td>
<td><input type="text" value="CSIT33254128" placeholder="..."></td>
</tr>
<tr>
<td>STUDENT FAMILY NAME</td>
<td><input type="text" value="Johny" placeholder="..."></td>
</tr>
<tr>
<td>STUDENT GIVEN NAME</td>
<td><input type="text" value="John Smith" placeholder="..."></td>
</tr>
<tr>
<td>UOW EMAIL</td>
<td><input type="text" value="johnsmith124@gmail.com" placeholder="..."></td>
</tr>
<tr>
<th colspan="2">ASSIGNMENT DETAILS</th>
</tr>
<tr>
<td>SUBJECT CODE</td>
<td><input type="text" value="" placeholder="CSIT..."></td>
</tr>
<tr>
<td>SUBJECT NAME</td>
<td><input type="text" value="Information Technology" placeholder="Introduction to..."></td>
</tr>
<tr>
<td>ASSIGNMENT NUMBER</td>
<td><input type="text" value="IT452" placeholder="..."></td>
</tr>
<tr>
<td>ASSIGNMENT DUE DATE</td>
<td><input type="text" value="" placeholder="../../...."></td>
</tr>
<tr>
<td>LAB TIME</td>
<td><input type="text" value="" placeholder="..."></td>
</tr>
</tbody>
</table>
</div>
</body>
</html>
Web Form 2

Script:
<!DOCTYPE html>
<html>
<head>
<title>Form 1</title>
<style>
body{
font-family: monospace;
}
.container {
padding-right: 20%;
padding-left: 20%;
margin-right: auto;
margin-left: auto;
}
.outer{
background-color: #131365;
margin-right: -15px;
margin-left: -15px;
display: inline-block;
}
.inner-50{
width: 50%;
float: left;
padding-left: 15px;
padding-right: 15px;
box-sizing: border-box;
}
.inner-50.left{
background-color: #a4c9e7;
}
.inner-50.right{
background-color: #131365;
color: white;
}
.inner-50 h2{
text-align: center;
}
.group{
margin-bottom: 20px;
padding-left: 15px;
padding-right: 15px;
text-align: center;
}
.group .control{
margin-top: 5px;
width: 100%;
height: 34px;
background-color: #fff;
background-image: none;
border: 1px solid #ccc;
border-radius: 4px;
margin-bottom: 20px;
}
.inner-group{
width: 100%;
display: inline-block;
text-align: left;
}
.stacked-group{
float: left;
width: 38%;
display: inline-block;
margin-right: 10px;
box-sizing: border-box;
}
.btn-success{
background-color: #c1c1c1;
color: #080808;
border-radius: 5px;
display: inline-block;
padding: 6px 12px;
margin-bottom: 0;
font-size: 14px;
font-weight: 400;
line-height: 1.42857143;
text-align: center;
white-space: nowrap;
vertical-align: middle;
-ms-touch-action: manipulation;
touch-action: manipulation;
cursor: pointer;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
background-image: none;
border: 1px solid transparent;
border-radius: 4px;
}
.checkbox-label{
display: inline-block;
vertical-align: middle;
}
.text-center{
text-align: center;
}
</style>
</head>
<body>
<div class="container">
<h1><b>Part 2: form 1</b></h1>
<form action="http://api/perform-search">
<div class="outer">
<div class="inner-50 left">
<h2>Choose Advanced Search Options</h2>
<p><label><input class="checkbox-label" type="checkbox"> Multi-Subject Resources</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Dissertation and Theses</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Current News Sources</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Books</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Arts & Humanities Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Business Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Education Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Engineering Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Health Sciences Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> International and Area Studies</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Life Sciences Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Library & Information Science Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Music / Performing Arts</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Physical Sciences/Math Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Social Science Articles</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Web Search Engines</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Dictionaries, Encyclopedias, etc.</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Image Search</label></p>
<p><label><input class="checkbox-label" type="checkbox"> Datasets</label></p>
</div>
<div class="inner-50 right">
<h2>Enter Advanced Search Terms</h2>
<div class="group">
<label>Keywords:</label>
<input class="control" type="text"/>
</div>
<div class="group">
<label>Title Words:</label>
<input class="control" type="text"/>
</div>
<div class="group">
<label>Author's Name:</label>
<br><br><br>
<div class="inner-group">
<div class="stacked-group">
<label>Last Name</label>
<input class="control" type="text" />
</div>
<div class="stacked-group">
<label>First Name</label>
<input class="control" type="text" />
</div>
<div class="stacked-group"
style="width: 60px;">
<label>Initial</label>
<input class="control" type="text" />
</div>
</div>
</div>
<div class="text-center">
<button class="btn-success" type="submit">Perform Search</button>
</div>
</div>
</div>
</form>
</div>
</body>
</html>
Web Form 3

Script:
<!DOCTYPE html>
<html>
<head>
<style>
.container {
padding-right: 20%;
padding-left: 20%;
margin-right: auto;
margin-left: auto;
}
.outer{
margin-right: -15px;
margin-left: -15px;
display: inline-block;
width: 100%;
}
.inner-50{
width: 50%;
float: left;
padding-left: 15px;
padding-right: 15px;
box-sizing: border-box;
}
.inner-50 .checkbox label input{
margin-right:10px;
}
.inner-50 .checkbox label{
font-weight: 600;
width: 100%;
display: inline-block;
}
.inner-50 small{
padding-left: 30px;
display: inline-block;
color: #514f4f;
}
.hr-bold{
font-weight: 600;
}
.group .control{
margin-top: 5px;
width: 100%;
height: 34px;
background-color: #fff;
background-image: none;
border: 1px solid #ccc;
border-radius: 4px;
margin-bottom: 20px;
}
.group textarea{
margin-top: 5px;
width: 100%;
background-color: #fff;
background-image: none;
border: 1px solid #ccc;
border-radius: 4px;
margin-bottom: 20px;
}
.group select{
width: auto !important;
}
.group{
width: 100%;
}
.col-2{
width: 16.66%;
}
.col-10{
width: 83.33%;
}
.btn-success{
background-color: #0fb3b3;
color: white;
border-radius: 5px;
display: inline-block;
padding: 6px 12px;
margin-bottom: 0;
font-size: 14px;
font-weight: 400;
line-height: 1.42857143;
text-align: center;
white-space: nowrap;
vertical-align: middle;
-ms-touch-action: manipulation;
touch-action: manipulation;
cursor: pointer;
-webkit-user-select: none;
-moz-user-select: none;
-ms-user-select: none;
user-select: none;
background-image: none;
border: 1px solid transparent;
border-radius: 4px;
}
</style>
</head>
<body>
<div class="container">
<h1><b>Part 3: form 2</b></h1>
<hr class="hr-bold">
<b>Select the type of Gift Card</b>
<hr>
<form action="http://pay-now">
<div class="outer">
<div class="inner-50">
<div class="checkbox">
<label><input type="radio" name="giftCard">Physical Gift Card</label>
<small> A traditional gift card that is personalised and delivered to the receipient's doorstep. It can be
used
both online and in-store.</small>
</div>
</div>
<div class="inner-50">
<div class="checkbox">
<label><input type="radio" name="giftCard">Digital Gift Card</label>
<small>Digital Cards are sent by email within minutes and can be redeemed both in stores and online</small>
</div>
</div>
</div>
<hr class="hr-bold">
<b>Personalise your Gift Card</b>
<hr>
<div class="group">
<label>Gift Card Value</label>
<input type="number" class="control" style="width: 200px;" />
<b>e.g., 100</b>
</div>
<hr>
<div class="outer">
<div class="inner-50">
<div class="group" style="display:inline-block">
<label style="width: auto;display: inline-block;margin-top: 10px;">Name</label>
<input type="text" class="control" style="max-width: 80%;float: right;"/>
</div>
<div class="group" style="display:inline-block">
<label style="width: auto;display: inline-block;margin-top: 10px;">To Email</label>
<input type="email" class="control" style="max-width: 80%;float: right;" />
</div>
<div class="group">
<label><b>Postal address</b></label>
<textarea rows="9"></textarea>
</div>
<div class="group">
<label><b>Custom greeting</b></label>
<textarea rows="9"></textarea>
</div>
</div>
<div class="inner-50">
<h2><b>Payment</b></h2>
<div class="group">
<label>Card Type</label>
<div>
<select class="control">
<option>VISA</option>
<option>MasterCard</option>
</select>
</div>
</div>
<div class="group">
<label>Card Number</label>
<div>
<input type="number" class="control" />
</div>
</div>
<div class="group">
<label>Valid to</label>
<div>
<select class="control">
<option>01</option>
<option>02</option>
<option>03</option>
<option>04</option>
<option>05</option>
<option>06</option>
<option>07</option>
<option>08</option>
<option>09</option>
<option>10</option>
<option>11</option>
<option>12</option>
</select>
<select class="control">
<option>2017</option>
<option>2018</option>
<option>2019</option>
<option>2020</option>
</select>
</div>
</div>
<div class="group">
<label>Name on card</label>
<input type="text" class="control" />
</div>
<div class="group">
<label>CVV2 Number</label>
<input type="number" class="control" style="width:inherit;" />
</div>
<button class="btn-success" type="submit">Buy Now</button>
</div>
</div>
<hr>
</form>
</div>
</body>
</html>
Send your request at realcode4you@gmail.com to get any HTML,CSS, JavaScript related project help
Comentários