Fun Quiz Game Using C Programming | C Programming Assignment Help
- realcode4you
- Sep 30, 2022
- 3 min read
Requirement Details
Make sure in this quiz it contains the following
selection (if or switch case),
repetition (for or/and while or/and do while)
at least one function with an array of struct in the input parameter list; the array of struct is declared in main (or another function) and this function must set values in the array of struct, and the calling function (main or other) must make use of the array of struct on return (you choose whether to pass by value or pass by address)
at least one function with a pointer to a variable of type char, int, float or double in the parameter list; the function must set the contents of the variable, and the calling function (main or other) must make use of the variable on return
your program needs several instances where the user must input commands from the keyboard in order to interact with the program
your program must loop to the start allowing the user to run through the program time after time (this is an independent requirement from the second point to use repetition).
Implementation
//This is a simple quiz test programmed in c
//The data of quiz is coming from quiz.csv file
//Once the quiz starts, here the user needs to answer each question one by one
//for each question, we display the question and then its four options
//now the user is prompted to input the answer (1, 2, 3 or 4 which represent option A, option B, option C or option D respectively)
//now once the user enters the answer, we check if the user entered answer is correct
//if answer is correct +4 marks are awarded
//if its incorrect -1 marks are awarded
//current score is always displayed in the screen
//after the test is done, the user is shown the final score he/she obtained from say 20 marks(since here we are only having 5 questions and each question has 4 marks so, 5*4 = 20)
//after that the user is asked whether he wants to restart the quiz (0, 1)
//here 1 means he wants to restart and 0 means he wants to exit
//include header files
#include <conio.h>
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
//Creating structure using struct keyword to declair the variables
typedef struct
{
char question[1024];
char optionA[1024];
char optionB[1024];
char optionC[1024];
char optionD[1024];
int answer;
} CHALLENGE;//defining the type "CHALLENGE" in form of structure, in simple terms it would represent our question, its 4 options and its answer.
//just prints the question along with its multiple choices
void printQuestion(CHALLENGE* question, int * questionNumber){
printf("Q%d. %s; \n\n", *questionNumber + 1, question->question);
printf("(1). %s; \n", question->optionA);
printf("(2). %s; \n", question->optionB);
printf("(3). %s; \n", question->optionC);
printf("(4). %s; \n\n", question->optionD);
}
//imports questions from quiz.csv file, here in the parameter list there is a pointer pointing to array of structures
void importQuestions(CHALLENGE* questions){
FILE* fp = fopen("quiz.csv", "r");
// Here we have taken size of
// array 1024 you can modify it
char buffer[1024];
int row = 0;
int column = 0;
while (fgets(buffer,
1024, fp)) {
column = 0;
char* value = strtok(buffer, ";");
CHALLENGE question;
while (value) {
if (column == 0) {
strcpy(question.question, value);
}
if (column == 1) {
strcpy(question.optionA, value);
}
if (column == 2) {
strcpy(question.optionB, value);
}
if (column == 3) {
strcpy(question.optionC, value);
}
if (column == 4) {
strcpy(question.optionD, value);
}
if (column == 5) {
question.answer = atoi(value);
}
value = strtok(NULL, ";");
column++;
}
questions[row] = question;
row++;
}
// Close the file
fclose(fp);
}
//it takes in questionNumber and then asks the question by printing it, then asking for user input and finally checking whether the entered answer is correct
int askQuestion(CHALLENGE* questions, int questionNumber){
int gotItRight = 0;
printQuestion(&questions[questionNumber], &questionNumber);
printf("\n");
printf("Enter Answer: ");
int userAnswer;
scanf("%d", &userAnswer);
if(userAnswer == questions[questionNumber].answer)
printf("Right Answer!!!"), gotItRight = 1;
else
printf("You got it wrong !\nCorrect answer is option number: %d\n\n", questions[questionNumber].answer);
getchar();
return gotItRight? 4: -1;
}
//set the exitGame variable a value of 0;
void setExitGame(int *exitGame){
*exitGame = 0;
return;
}
// Driver Code
int main()
{
start:
int restart = 0;
CHALLENGE* questions = malloc(1000 * sizeof *questions);
importQuestions(questions);
int exitGame;
setExitGame(&exitGame);
int totalScore = 0;
int i = 1;
printf("Lets start the quiz!!!\n\n");
printf("You will be asked 5 questions, for correct answer you get +4 and for incorrect you get -1");
printf("\nPress Any Key to Continue\n");
getchar();
while(!exitGame){
system("cls");
printf("Your Current Score is: %d\n\n", totalScore);
totalScore += askQuestion(questions, i-1);
printf("\nNow Current Score is: %d\n", totalScore);
if(i == 5)
exitGame = 1;
i++;
printf("\nPress Any Key to Continue\n");
getchar();
}
system("cls");
printf("Your got %d, out of %d\n", totalScore, 20);
printf("Do you want to restart(0/1)?\n");
scanf("%d", &restart);
if(restart)
goto start;
return 0;
}
Output:

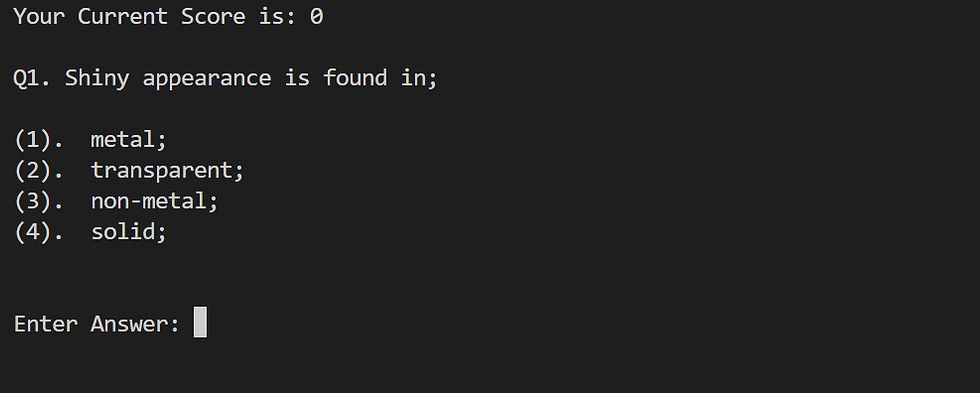
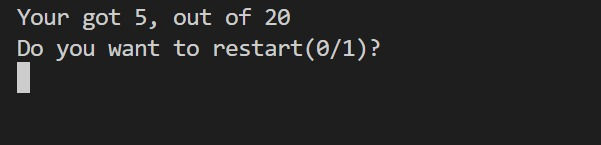
Go get other C programming related help you can send your requirement details at:
realcode4you@gmail.com
Comments